Open Folder in Visual Studio Code and write a C++ program
- G-code is Numerical Control (NC) programming language. It is used in Computer Aided Manufacturing (CAM) to control automated machines. G-code language tells a machine's controller which tool to use, where to move, how fast to move, and what path to follow. G-Code is also use in 3D printing.
- In this video I am going to show, How to Set Up C Development With Visual Studio Code on Windows 10. We will use MinGW with VS code as our compiler and deb.
- Close Visual Studio Code if it’s currently open. Then Launch Visual Studio Code. Choose File -> Open Folder…
- Create a brand new folder at any location of your choosing. In this example, the new folder will be called coding.
- In the main VS Code editor, click on File -> New File, and then press Ctrl+S to save it as “code.cpp”.
- Install C++ extension for VS Code.
Set compilerPath
It is possible now to run Visual Studio code (and other graphical interface programs) inside WSL (by installing XFCE and with the help of and X Server) or, better, to use remote development (see. Visual Studio Code (VS Code) is a free, cross-platform, and lightweight source-code editor developed by Microsoft for Windows, Linux, and Mac operating systems. It is a source-code editor while Visual Studio is an IDE (integrated development environment).
- Press Ctrl+Shift+P, start typing “C/C++” and then choose Edit Configurations (JSON) from the list of suggestions. VS Code creates a file called c_cpp_properties.json and populates it with default settings.
- Find the
compilerPath
setting and paste in the full path name of g++.exe in the Mingw-w64 bin folder you have just appended to the Path variable. (C:Mingw-w64mingw32bing++.exe
, notice how you have to do double “” due to json convention)
- Set
intelliSenseMode
togcc-x64
. - Your
c_cpp_properties.json
file should look like this:
Create a build task
- Next, Press Ctrl+Shift+P again and start typing “task” and choose Tasks: Configure Default Build Task from the list of suggestions, then choose Create tasks.json file from template. Then choose MSBuild. VS Code creates a default tasks.json file in the editor.
- Find the
command
setting and change it tog++
. - Change
args
setting to[ '-g', '-o', 'a.exe', 'code.cpp']
- Your
tasks.json
should look like this:
Configure debug settings
Next, we’ll configure VS Code so that it launches the gdb debugger properly.- Click Debug -> Open Configurations, and then choose C++ (GDB/LLDB) from the list of suggestions. VS Code creates a default launch.json file in the editor.
- Find the
program
setting and delete everything except for the last part${workspaceFolder}/a.exe
. - Find the
miDebuggerPath
and point it to the gdb file in your Mingw-w64 bin folder, i.e.C:Mingw-w64mingw32bingdb.exe
- Your complete
launch.json
file should look like this:
Set breakpoint and debug
- Go to code.cpp in the editor, write your C++ program and Press Ctrl+Shift+B and then Enter to compile.
- Then set a break point and Press F5 to debug.
Additional bonus settings
- After any changes to source code, always remember to Ctrl+Shift+B to build before you run debug. If you want F5 to always rebuild the code by default, insert this right below the line of
program
setting in thelaunch.json
file. - Ideally you want the debug output to be on a separate console. In
launch.json
, findexternalConsole
setting and change it totrue
.
Visual Studio Code (VSCode) is a lightweight, open-source code editor and available on Linux, Mac OSX, and Windows. One of the key features of Visual Studio Code is its great debugging support for Node.js (JavaScript and TypeScript) and another feature is to run Tasks(Grunt, Gulp, MSBuild…etc.) from the IDE. If you are new to VSCode, I would recommend to see following video which shows how to debug Node.js app, put break-point and use watch window, use environment variable in Visual Studio Code editor:
Used software/tools for this article:
Windows 10
Node 4.4.0
Visual Studio Code 0.10.11 Beta
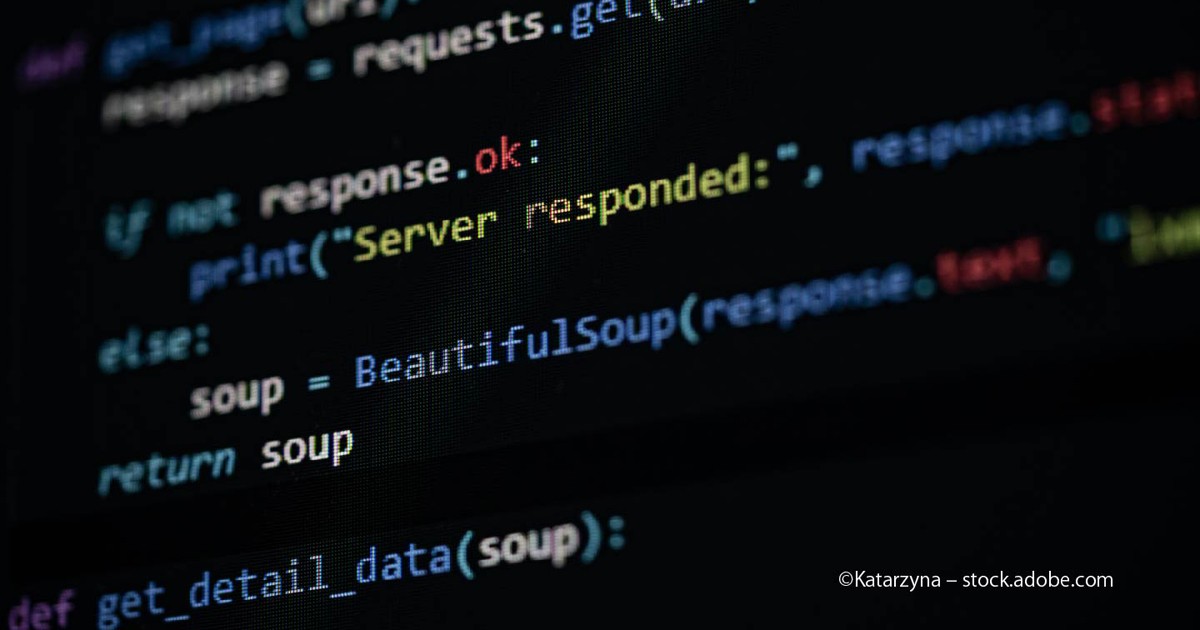
Debugging:
Here is the content of the above video:
Go to project directory in command prompt and use following command to open VSCode
Create a new file app.js and use following code
Setup Launch configuration:
Click Debug icon > Configure gear icon on the Debug view top bar > Select debug environment: Node.
It will generate a launch.json
in the Debug view > Select “Launch” in dropdown >F5 or click green arrow to start debug session.
You will get “Hello World” output in debug console.
You can put break-point and evaluate expression in watch window.
For more information related to debugging, see official notes.
Let’s update code to use environment variable:
See More Results
Now you will get the following result:
Use G++ In Visual Studio
Tasks:
Let’s come on the main point of this post. Before we get started, it is important to understand how Tasks are executed in VSCode. Let’s take Gulp task as an example. Here is the complete Video.
1. Goto project directory and install gulp package. It is recommended to install globally and use package.json file but for simplicity, to understand VSCode, we install it locally. Run following command to install gulp package.
2. Open VSCode, create gulpfile.js and use following code
3. Press F1 to open the Command Palette > type “Configure Task Runner” > Enter to select
This will create a sample “tasks.json” file in the .vscode folder. The initial file has a large number of examples within it. Use following for Gulp hello task
4. As this is the only task in the file, you can execute it by simply pressing Ctrl+Shift+B (Run Build Task). You will get Hello World in the output window.
5. Add one more task in gulpfile.js which uses environment variable:
6. Add one more task in task.json in tasks array
C Programming In Vs Code
7. F1 > Run Task > Select envtask > Enter.
You will get following result
8. tasks.json allows to set environment variable, it is in options. Here is the complete view of tasks.json:
9. F1 > Run Task > Select envtask > Enter.
You will get following result
Conclusion:
We have seen how VSCode’s built-in debugger helps accelerate edit, compile and debug loop and how to run Gulp tasks and pass environment variable in it. Both are the important features of VSCode. If you are .NET guy or Visual Studio fan, definitely you will like VSCode for front-end development especially on non-Windows platform. Enjoy VSCode !!
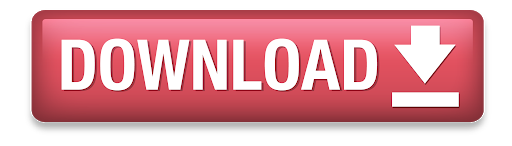